In this tutorial, we will learn how we can secure application secrets using Jasypt. Also, we will see one practical example using the Spring Boot application.
Jasypt Overview
Jasypt (Java Simplified Encryption) is a library using which we can encrypt and decrypt our application secrets. Instead of hardcoding the secrets in our application configuration(application.properties / application.yaml), we will encrypt the secrets using the Jasypt library and store the encrypted value inside the application.properties / application.yaml instead of plain text. Our application will decrypt the value and use the original value wherever required.
High-Level Steps
- Add Jasypt spring boot starter dependency in the pom.xml of the application.
- Encrypt the values using Jasypt CLI commands or by using the Jasypt maven plugin.
- Store the encrypted values instead of the original plain text inside the application.properties / application.yaml file.
- Read the properties using @Value Spring annotation which will inject the decrypted values into the respective fields.
- Use the fields wherever required in our application class.
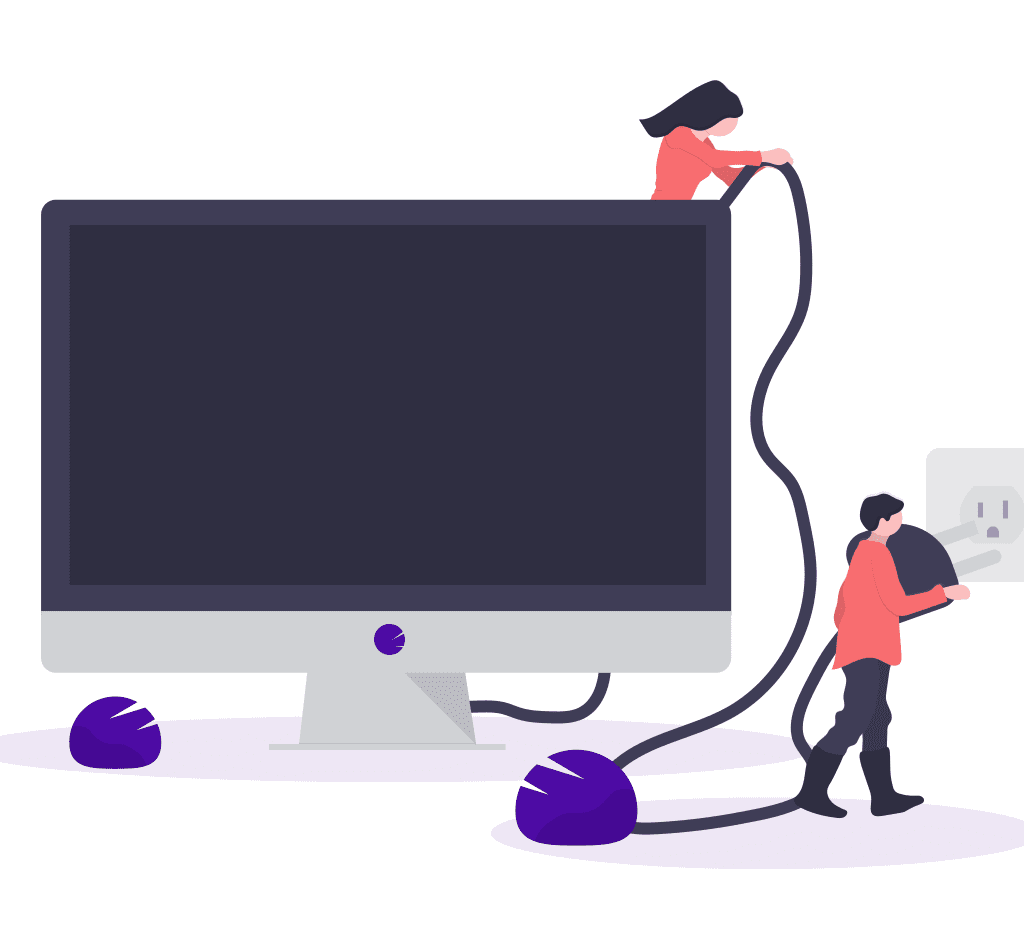
Encrypting & Decrypting Secrets
Using Jasypt CLI commands
- Download the Jasypt jar file from the maven repository and place it inside any folder on the system.
- Start the terminal/command prompt and change the path to the folder containing the Jasypt jar file.
Steps to Encrypt Secret
This is required to generate the encrypted value so that we can store the generated value inside the application.properties / application.yaml file of the application.
1. Encrypt the secret using the below command
java -cp jasypt-1.9.3.jar org.jasypt.intf.cli.JasyptPBEStringEncryptionCLI input="Password@123" password=SomeStrongEncryptionKey algorithm=PBEWITHHMACSHA512ANDAES_256 ivGeneratorClassName=org.jasypt.iv.RandomIvGenerator
CMDWhere:
- jasypt-1.9.3.jar is the name of the jar file downloaded from the Maven repository.
- JasyptPBEStringEncryptionCLI is the name of the class responsible for performing encryption.
- Password@123 is the secret that we want to encrypt.
- SomeStrongEncryptionKey is the key using which encryption will be performed.
- PBEWITHHMACSHA512ANDAES_256 is the name of the algorithm.
- RandomIvGenerator is the name of the IV generator that will produce different outputs with each run of the encryption even if we use the same encryption key.
2. Assign the encrypted value to the required property inside the application.properties / application.yaml file.
Syntax: propertyName=ENC(valueObtainedFromStep1)
some.encrypted.property=ENC(ZbDLXoFgnhNTmluLzjZBu/Bq17+pSnuvSyLr23b7RBfBukBXBfWRFQx7626OkQL3)
CMDSteps to Decrypt Secret
This is only required if we want to see the decrypted value manually.
1. Decrypt the secret using the below command
java -cp jasypt-1.9.3.jar org.jasypt.intf.cli.JasyptPBEStringDecryptionCLI input="ZbDLXoFgnhNTmluLzjZBu/Bq17+pSnuvSyLr23b7RBfBukBXBfWRFQx7626OkQL3" password=SomeStrongEncryptionKey algorithm=PBEWITHHMACSHA512ANDAES_256 ivGeneratorClassName=org.jasypt.iv.RandomIvGenerator
CMDWhere:
- jasypt-1.9.3.jar is the name of the jar file downloaded from the maven repository.
- JasyptPBEStringDecryptionCLI is the name of the class responsible for performing decryption.
- ZbDLXoFgnhNTmluLzjZBu/Bq17+pSnuvSyLr23b7RBfBukBXBfWRFQx7626OkQL3 is the secret that we want to decrypt. It is the same value that was obtained after performing the encryption.
- SomeStrongEncryptionKey is the key using which decryption will be performed. It should be the same key that was used during the encryption.
- PBEWITHHMACSHA512ANDAES_256 is the name of an algorithm that was used during the encryption.
- RandomIvGenerator is the name of the IV generator that was used during the encryption.
Using Jasypt Maven Plugin
- Add jasypt-maven-plugin inside the build > plugins section of pom.xml.
<plugin>
<groupId>com.github.ulisesbocchio</groupId>
<artifactId>jasypt-maven-plugin</artifactId>
<version>3.0.5</version>
</plugin>
JavaSteps to Encrypt Secret
This is required to generate the encrypted value so that we can store the generated value inside the application.properties / application.yaml file of the application.
1. Encrypt the secret using the below command
mvn jasypt:encrypt-value -Djasypt.encryptor.password=Password@123 -Djasypt.plugin.value=SomeStrongEncryptionKey
CMDWhere:
- Password@123 is the secret that we want to encrypt.
- SomeStrongEncryptionKey is the key using which encryption will be performed.
- PBEWITHHMACSHA512ANDAES_256 is the name of the algorithm.
- RandomIvGenerator is the name of the IV generator that will produce different outputs with each run of the encryption even if we use the same encryption key.
2. Assign the encrypted value to the required property inside the application.properties / application.yaml file.
Syntax: propertyName=ENC(valueObtainedFromStep1)
some.encrypted.property=ENC(ZbDLXoFgnhNTmluLzjZBu/Bq17+pSnuvSyLr23b7RBfBukBXBfWRFQx7626OkQL3)
CMDSteps to Decrypt Secret
This is only required if we want to see the decrypted value manually.
1. Decrypt the secret using the below command
mvn jasypt:decrypt-value -Djasypt.encryptor.password=SomeStrongEncryptionKey -Djasypt.plugin.value=ZbDLXoFgnhNTmluLzjZBu/Bq17+pSnuvSyLr23b7RBfBukBXBfWRFQx7626OkQL3
CMD- ZbDLXoFgnhNTmluLzjZBu/Bq17+pSnuvSyLr23b7RBfBukBXBfWRFQx7626OkQL3 is the secret that we want to decrypt. It is the same value that was obtained after performing the encryption.
- SomeStrongEncryptionKey is the key using which decryption will be performed. It should be the same key that was used during the encryption.
- PBEWITHHMACSHA512ANDAES_256 is the default algorithm that will be used for decryption.
- RandomIvGenerator is the name of the default IV generator that will be used during the decryption.
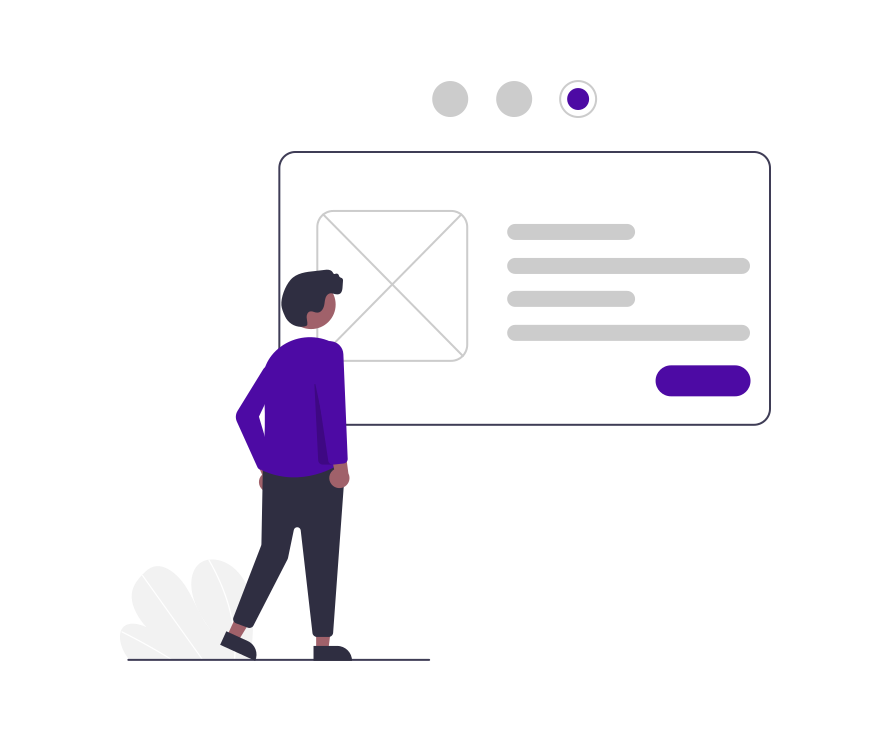
Spring Boot Example
In this example, we have stored the encrypted value inside the application.properties of our application. We are reading this value inside our controller class and then sending the decrypted value inside the API call response.
# decoded value of some.encrypted.property is Password@123
some.encrypted.property=ENC(ZbDLXoFgnhNTmluLzjZBu/Bq17+pSnuvSyLr23b7RBfBukBXBfWRFQx7626OkQL3)
application.properties@RestController
public class SpringBootJasyptController {
@Value("${some.encrypted.property}")
private String someProperty;
@GetMapping("/getDecryptedValue")
public String sendDecryptedValue() {
return "Decrypted value: " + someProperty;
}
}
JavaSource Code
The source code for this example can be found on GitHub. Link: click here
- The commands for building the project, and running an application/test cases need to run inside the root folder of this project i.e. inside the spring-boot-jasypt folder.
- The value of jasypt.encryptor.password should be the same key using which we generated the encrypted value.
How to Build Maven Project
Run the below command to build the maven project.
mvn clean install -Djasypt.encryptor.password=SomeStrongEncryptionKey
CMDHow to Run Application
Start the application using any of the commands mentioned below.
Using Maven
mvn spring-boot:run -Dspring-boot.run.arguments=--jasypt.encryptor.password=SomeStrongEncryptionKey
CMDFrom JAR File
Create a jar file using by building the maven project and then execute
java -jar target/spring-boot-jasypt-0.0.1-SNAPSHOT.jar --jasypt.encryptor.password=SomeStrongEncryptionKey
CMDHow to Verify Output
Start the application and call the controller endpoint.
Send an HTTP GET request to the ‘/getDecryptedValue’ endpoint using any of the two methods.
Browser or Rest Client
http://localhost:8080/getDecryptedValue
BroswercURL
curl --request GET 'http://localhost:8080/getDecryptedValue
TerminalHow to Run Test Cases
Run the test cases using any of the commands mentioned below.
Run All Test Cases
mvn test -Djasypt.encryptor.password=SomeStrongEncryptionKey
CMDRun Individual Test Case
mvn -Dtest=SpringBootJasyptControllerTest test -Djasypt.encryptor.password=SomeStrongEncryptionKey
CMDmvn -Dtest=SpringBootJasyptApplicationTests test -Djasypt.encryptor.password=SomeStrongEncryptionKey
CMDSummary
We understood how we can secure our application secrets using Jasypt. There are multiple ways using which we can encrypt the values. Also, we practically implemented the same inside our Spring Boot application.
Add a Comment