Understand how to handle URL trailing slash changes in Spring Boot 3.
Context
If you are upgrading your web application to Spring Boot 3 then you need to review the changes that were introduced related to URL matching.
Earlier trailing slash match configuration for URLs was set to true by default but this configuration option has been as a part of Spring Framework 6.x and it’s default value is set to false due to which you may observe HTTP 404 error for the requests that you are receiving.
Example
GET /some/greeting
Example 1GET /some/greeting/
Example 2In Example 2, you will see we have one additional slash at the end of the URL. Before Spring Framework 6.x, the above two URLs were treated as same but starting with Spring Boot 3.x, these URLs will be treated as different URLs and this will result in HTTP 404 error.
Ways to Handle
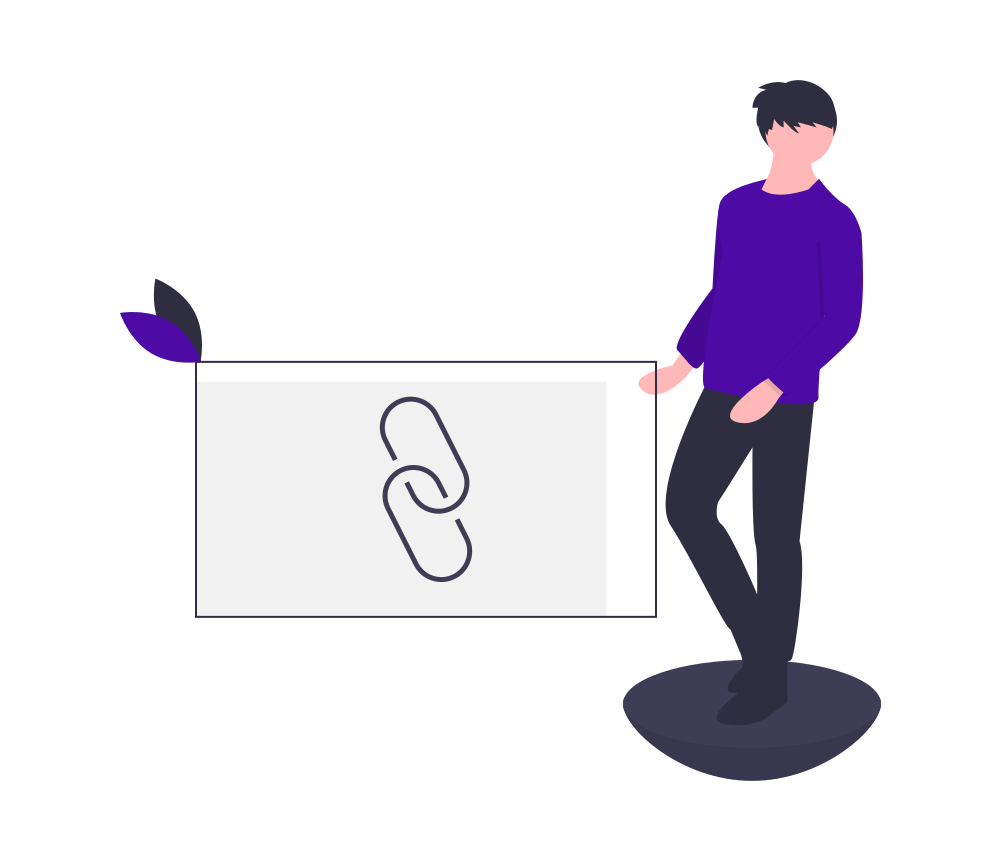
Adding URLs in Controller Handler
In this method, you need to add an additional route explicitly on the controller handler. This means you need to add both URLs for all the controller endpoints.
@GetMapping({"/some/greeting", "/some/greeting/"})
public String sendGreetings() {
return "Hello User";
}
JavaIn the above example, you can see we have explicitly specified two URLs for our endpoint.
Update Global Spring MVC Configuration
In this method, instead of adding additional URLs, we will change the default configuration related to trailing slash match and set it to true. This will restore the old configuration that was present before Spring Framework 6.x. After updating this configuration the URLs with trailing slash and URLs without trailing slash will be treated as same.
We can update this configuration in the case of Spring MVC as well as in the case of Spring WebFlux.
Spring MVC
@Configuration
public class WebConfiguration implements WebMvcConfigurer {
@Override
public void configurePathMatch(PathMatchConfigurer configurer) {
configurer.setUseTrailingSlashMatch(true);
}
}
JavaSpring WebFlux
@Configuration
public class WebConfiguration implements WebFluxConfigurer {
@Override
public void configurePathMatching(PathMatchConfigurer configurer) {
configurer.setUseTrailingSlashMatch(true);
}
}
JavaSummary
When upgrading to Spring Boot 3, review URL matching changes: trailing slash match config default is now false, so update your configurations to avoid HTTP 404 errors.
Overriding Spring MVC Configuration did not work. I had to add the additional controller value. Also note that your syntax for it is incorrect. It does not accept comma separated Strings. It takes a String array. Something like…
@GetMapping({ “/some/greeting”, “/some/greeting/”})
Thank you for your comment. I’ve rectified the issue with the @GetMapping annotation.
Regarding Spring MVC configuration, it is working fine. I have confirmed the same using the latest version of Spring Boot(3.1.5). It’s worth noting that the ‘setUseTrailingSlashMatch’ method has been deprecated.