It’s crucial for Java developers to comprehend Java arrays. Arrays are also the foundation of many data structures. We will go through a few key questions regarding java arrays in this tutorial.
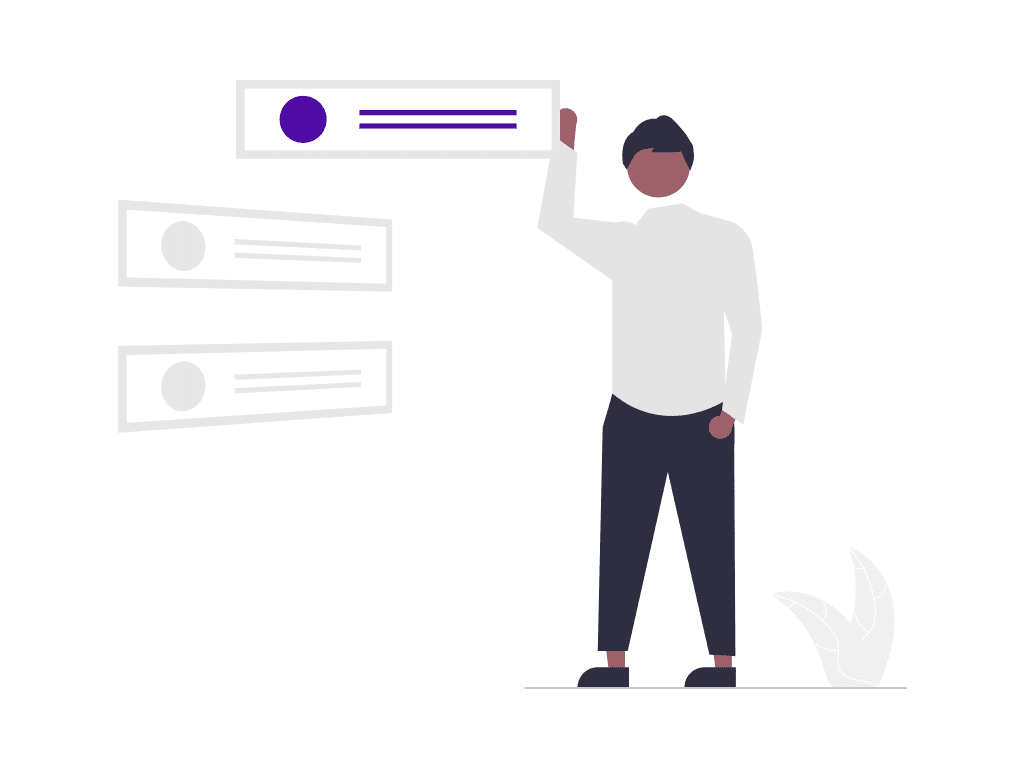
Can we create an array of size 0 in Java?
Yes, we can have an array with size 0. In such cases, we don’t get any compile time or runtime error.
Example
while running a java program, if we don’t pass any command line arguments, in that case, we don’t get any errors.
public class Arrays {
public static void main(String main[]) {
int[] x = new int[0]; // Valid
}
}
JavaWhat happens if we try to create an array with a negative size?
If we try to create an array with some negative integer value then we will get a runtime exception saying NegativeArraySizeException. Here, the job of the compiler is only to check whether the array size is of type integer or not.
Example
public class Arrays {
public static void main(String main[]) {
int[] x = new int[-3]; // Invalid
}
}
JavaD:\Java>javac Arrays.java
D:\Java>java Arrays
Exception in thread "main" java.lang.NegativeArraySizeException: -3
at Arrays.main(Arrays.java:3)
CMDWhich data types are allowed for specifying the array size?
To specify the array size, the allowed data types are byte, short, char, and int. If we try to specify the array size using any other data type then we will get a compile-time error stating ‘possible lossy conversion’.
Example
public class Arrays {
public static void main(String main[]) {
int[] a = new int[3]; // Valid
int[] b = new int['a']; // Valid
byte byteSize = 20;
int[] c = new int[byteSize]; // Valid
short shortSize = 30;
int[] d = new int[shortSize ]; // Valid
int[] e = new int[10l]; // Invalid
}
}
JavaD:\Java>javac Arrays.java
Arrays.java:13: error: incompatible types: possible lossy conversion from long to int
int[] e = new int[10l]; // Invalid
^
1 error
CMDWhat is the maximum size of an array?
In arrays, the maximum allowed size is 2,147,483,647 which is the maximum value of the int data type. If we try to specify an integer value greater than 2,147,483,647 then we will get a compiler time error stating ‘integer number too large’.
Even in case of maximum allowed value, we may get a runtime exception if sufficient heap memory is not available.
Example
public class Arrays {
public static void main(String main[]) {
int[] a = new int[2147483647]; // Valid
int[] b = new int[2147483648]; // Invalid
}
}
JavaD:\Java>javac Arrays.java
Arrays.java:5: error: integer number too large
int[] b = new int[2147483648]; // Invalid
^
1 error
CMDWhat will be the output of the length variable in the case of a multidimensional array?
In the case of a multidimensional array, the length variable represents only the base size but not the total size of an array. There is no direct way to specify the total length of multidimensional arrays.
Example
public class Arrays {
public static void main(String main[]) {
int[] a = new int[3];
System.out.println("1x1 Array Length: " + a.length);
int[][] b = new int[2][2];
System.out.println("2x2 Array Length: " + b.length);
int[][][] c = new int[3][3][3];
System.out.println("3x3x3 Array Length: " + c.length);
}
}
JavaD:\Java>javac Arrays.java
D:\Java>java Arrays
1x1 Array Length: 3
2x2 Array Length: 2
3x3x3 Array Length: 3
CMDWhat types of elements are allowed in different types of arrays?
The following types of elements are allowed in the case of different types of arrays:
-
Primitive Arrays – Any type of element which can be implicitly promoted to the declared type can be added.
-
Object Type Arrays – Either declared type or its child class objects can be added.
-
Abstract Class Type Arrays – Child class objects can be added.
-
Interface Type Arrays – Implementation class objects are allowed.
Can we assign a two-dimensional array to a one-dimensional array?
In place of a one-dimensional int array, we should provide a one-dimensional array only. Whenever we are assigning one array, dimensions must match or else we will get a compile-time error,
public class Arrays {
public static void main(String main[]) {
int[] a = new int[3];
int[] b = new int[5];
a = b; // Valid
int[][] c = new int[2][2];
a = c; // Invalid
}
}
JavaD:\Java>javac Arrays.java
Arrays.java:10: error: incompatible types: int[][] cannot be converted to int[]
a = c; // Invalid
^
1 error
CMDTop Picks for Learning Java
Explore the recommended Java books tailored for learners at different levels, from beginners to advanced programmers.
Disclaimer: The products featured or recommended on this site are affiliated. If you purchase these products through the provided links, I may earn a commission at no additional cost to you.
- All Levels Covered: Designed for novice, intermediate, and professional programmers alike
- Accessible Source Code: Source code for all examples and projects are available for download
- Clear Writing Style: Written in the clear, uncompromising style Herb Schildt is famous for
Head First Java: A Brain-Friendly Guide
- Engaging Learning: It uses a fun approach to teach Java and object-oriented programming.
- Comprehensive Content: Covers Java's basics and advanced topics like lambdas and GUIs.
- Interactive Learning: The book's visuals and engaging style make learning Java more enjoyable.
Modern Java in Action: Lambdas, streams, functional and reactive programming
- Latest Java Features: Explores modern Java functionalities from version 8 and beyond, like streams, modules, and concurrency.
- Real-world Applications: Demonstrates how to use these new features practically, enhancing understanding and coding skills.
- Developer-Friendly: Tailored for Java developers already familiar with core Java, making it accessible for advancing their expertise.
- Java Essentials: Learn fundamental Java programming through easy tutorials and practical tips in the latest edition of the For Dummies series.
- Programming Basics: Gain control over program flow, master classes, objects, and methods, and explore functional programming features.
- Updated Coverage: Covers Java 17, the latest long-term support release, including the new 'switch' statement syntax, making it perfect for beginners or those wanting to brush up their skills.
Add a Comment