This blog post provides a step-by-step guide on how to customize the 404 Not Found API response in Spring Boot using the NoHandlerFoundException. By creating a custom error response object and exception handler, you can provide more informative error messages to your API clients, improving their overall experience.
Introduction
When building a RESTful API using Spring Boot, developers may encounter a “404 Not Found” error when a client requests a resource that does not exist. In this case, the server does not have a handler to process the request, and it responds with a default error page. However, developers may want to customize the 404 response to provide more informative error messages to clients. Fortunately, Spring Boot provides a way to customize the 404 error response using the NoHandlerFoundException class.
Implementation
Step 1: Create a Custom Error Response Object
The first step in customizing the 404 error response is to create a custom error response object that will hold the error details. In this case, we will create a class called “ApiErrorResponse” with two fields: “code” to hold the error code and “message” to hold the error message.
public class ApiErrorResponse {
private int code;
private String message;
public ApiErrorResponse(int code, String message) {
this.code = code;
this.message = message;
}
public String getMessage() {
return message;
}
public int getCode() {
return code;
}
}
ApiErrorResponse.javaIn the example above, we’ve created a class called ApiErrorResponse with two fields, code, and message. We’ve also created a constructor that takes a code parameter to set the code field and a message parameter to set the message field. You can add more fields in the above class based on the details that you want to send to your API consumer like timestamp and so on.
Step 2: Create a Custom Exception Handler
The next step is to create a custom exception handler to handle the NoHandlerFoundException and return a JSON response with the custom error message.
import jakarta.servlet.http.HttpServletRequest;
import org.springframework.http.HttpStatus;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.servlet.NoHandlerFoundException;
@ControllerAdvice
public class ApiExceptionHandler {
@ExceptionHandler({NoHandlerFoundException.class})
public ResponseEntity<ApiErrorResponse> handleNoHandlerFoundException(
NoHandlerFoundException ex, HttpServletRequest httpServletRequest) {
ApiErrorResponse apiErrorResponse = new ApiErrorResponse(404, "Resource not found");
return ResponseEntity.status(HttpStatus.NOT_FOUND).contentType(MediaType.APPLICATION_JSON).body(apiErrorResponse);
}
}
ApiExceptionHandler.javaIn the example above, we’ve created a class called ApiExceptionHandler and annotated it with @ControllerAdvice.
We’ve created a method called handleNoHandlerFoundException(), which takes a NoHandlerFoundException parameter and returns a ResponseEntity with the custom error response object and HTTP status code.
Step 3: Add @EnableWebMvc Annotation
Add @EnableWebMvc annotation on top of your application main class or if you have any class annotated with @Configuration, you can add @EnableWebMvc annotation on top of that class.
Step 4: Update application.properties
The next step is to throw an exception if no handler mapping is found by adding the following code to your application.properties file:
spring.mvc.throw-exception-if-no-handler-found=true
application.propertiesStep 5: Test Using Some Invalid URL
Test the changes by sending a request to your application using some invalid URL that does not exist.
curl --location 'localhost:8080/some/invalid/url'
shellscriptThe below image shows how the customized error response will look like post the customization changes that we did inside the Spring Boot application.
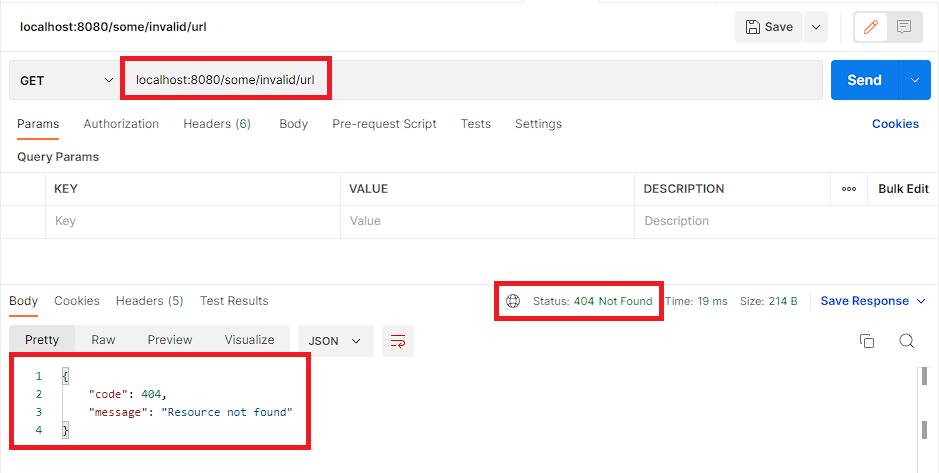
Conclusion
In this blog post, we learned how to customize the 404 Not Found API response using the NoHandlerFoundException class in Spring Boot. By following these steps, you can provide more informative error messages to your API clients and improve their overall experience.
Bonus
Curious to Learn Something New?
Check out how to handle URL trailing slash changes in Spring Boot 3.
Add a Comment