Understand how we can add tags in server requests metrics using Spring web MVC tags provider. This is useful for adding tags in application endpoint metrics. These tags will be added as additional tags on top of the default tags that are provided by the framework.
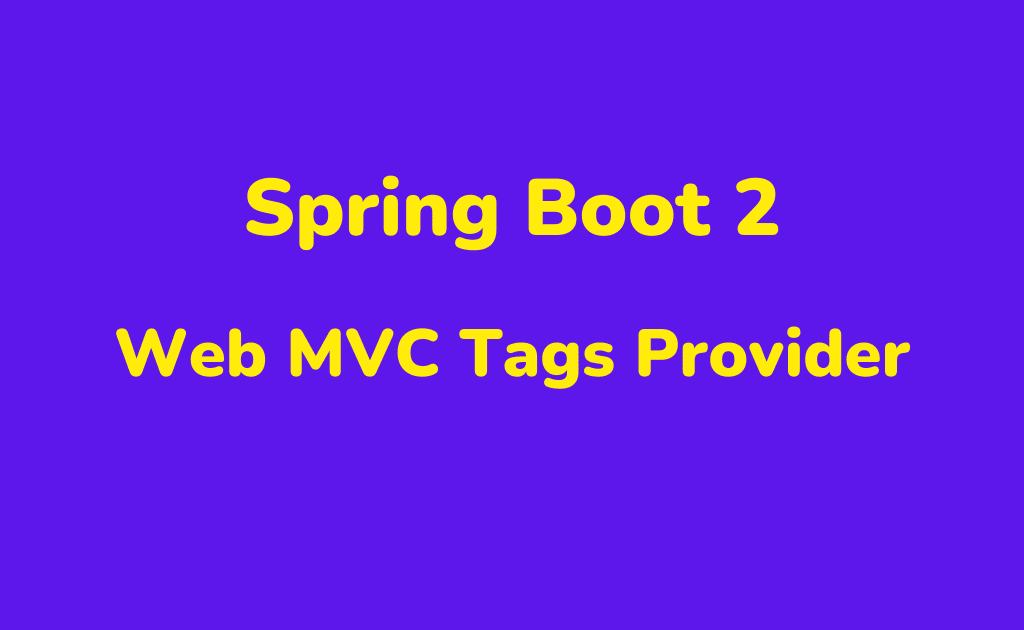
Context
When we add Spring Boot Starter Actuator dependency in our Spring Boot application, we can access the metrics for our application endpoints via the actuator metrics endpoint. In order to access the metrics data, we need to send the GET request specifying the name of metrics we are interested in i.e. in our case server requests metrics.
curl --request GET 'http://localhost:8080/actuator/metrics/http.server.requests'
ShellScriptBy default, metrics are available with the name ‘http.server.requests’. This name can be customized by setting one property in the application.properties file.
When we send the request to the above endpoint, by default, we get the following tags in the output:
- exception: any exceptions that were encountered during the request processing
- method: HTTP methods
- uri: endpoints for which we received the requests
- outcome: whether the request was processed successfully or not
- status: HTTP status code
Advantages
When we add our custom tags, we can utilize this information to create charts and dashboards displaying information at a more granular level.
Example: For each request, we can add a location tag highlighting the region from where we have received a request. Based on this information, we can create graphs and charts and see from what all regions we are receiving major traffic.
Adding Custom Tags
Note:
Below mentioned steps works only in case of Spring Boot 2.x. For Spring Boot 3.x, kindly refer to article Spring Boot 3: How To Add Tags in Server Requests Metrics.
If we want to add our own tags then we can do it with the help of Web MVC Tags Provider. These tags will override the default tags that are provided by the framework.
Steps:
- Create a class that implements the WebMvcTagsProvider interface.
- Provide implementation for the getTags method wherein we can add our own tags.
- Register this class as Spring Bean using @Component annotation.
import io.micrometer.core.instrument.Tag;
import io.micrometer.core.instrument.Tags;
import org.springframework.boot.actuate.metrics.web.servlet.WebMvcTagsProvider;
import org.springframework.stereotype.Component;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
/**
* The type Bootcamp to prod web mvc tags provider.
* Useful for overriding server metrics. This will override the default tags provided by Spring Framework.
*/
@Component
public class BootcampToProdWebMvcTagsProvider implements WebMvcTagsProvider {
@Override
public Iterable<Tag> getTags(HttpServletRequest request, HttpServletResponse response, Object handler, Throwable exception) {
var tags = Tags.empty();
// Optional tag which will be present in metrics only when the condition is evaluated to true
if (request.getParameter("user") != null) {
tags = tags.and(Tag.of("user", request.getParameter("user")));
}
// Custom tag which will be present in all the server requests metrics
tags = tags.and(Tag.of("tag", "value"));
return tags;
}
@Override
public Iterable<Tag> getLongRequestTags(HttpServletRequest request, Object handler) {
return null;
}
}
JavaWe have control over a request, response, and exception object. Based on this, we can add our own tags.
In the above example, we have added two tags “user” and “tag” wherein the “user” tag is optional and will be added to metrics only if we are receiving requests with the query parameter “user” whereas the “tag” tag will be added to metrics for the all the requests that we have received.
Output:
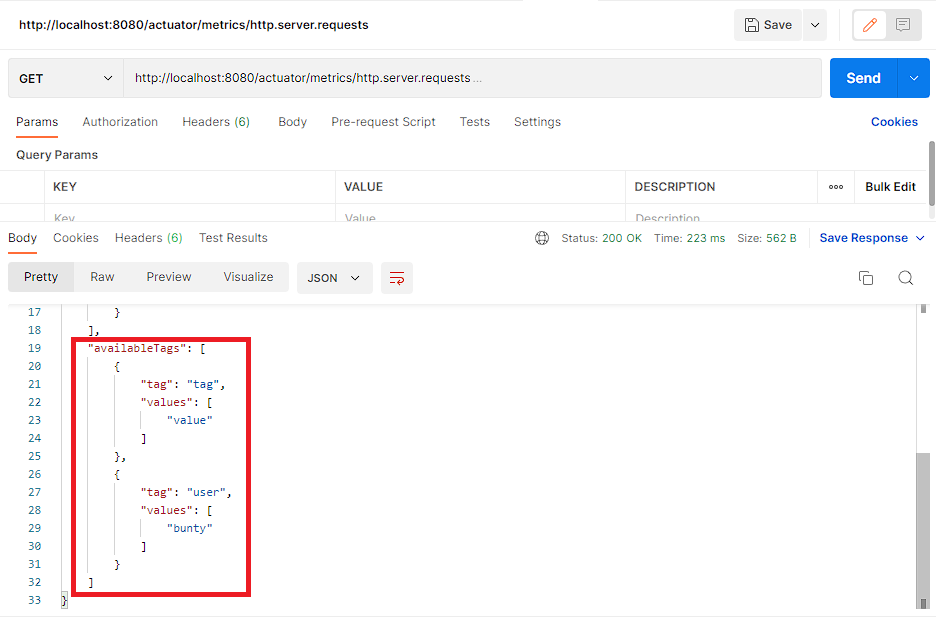
Source Code
The source code for this example can be found on GitHub. Link: click here
Learn More
Curious to Learn Something New?
Check out how we can override the default tags in Spring Boot 2.
Add a Comment